How to use `folium.colormap` in choropleths
By Deepnote team
•Updated on November 23, 2023
Discover the art of creating informative and visually appealing maps using `folium.colormap`. This guide covers various techniques for effective data representation.
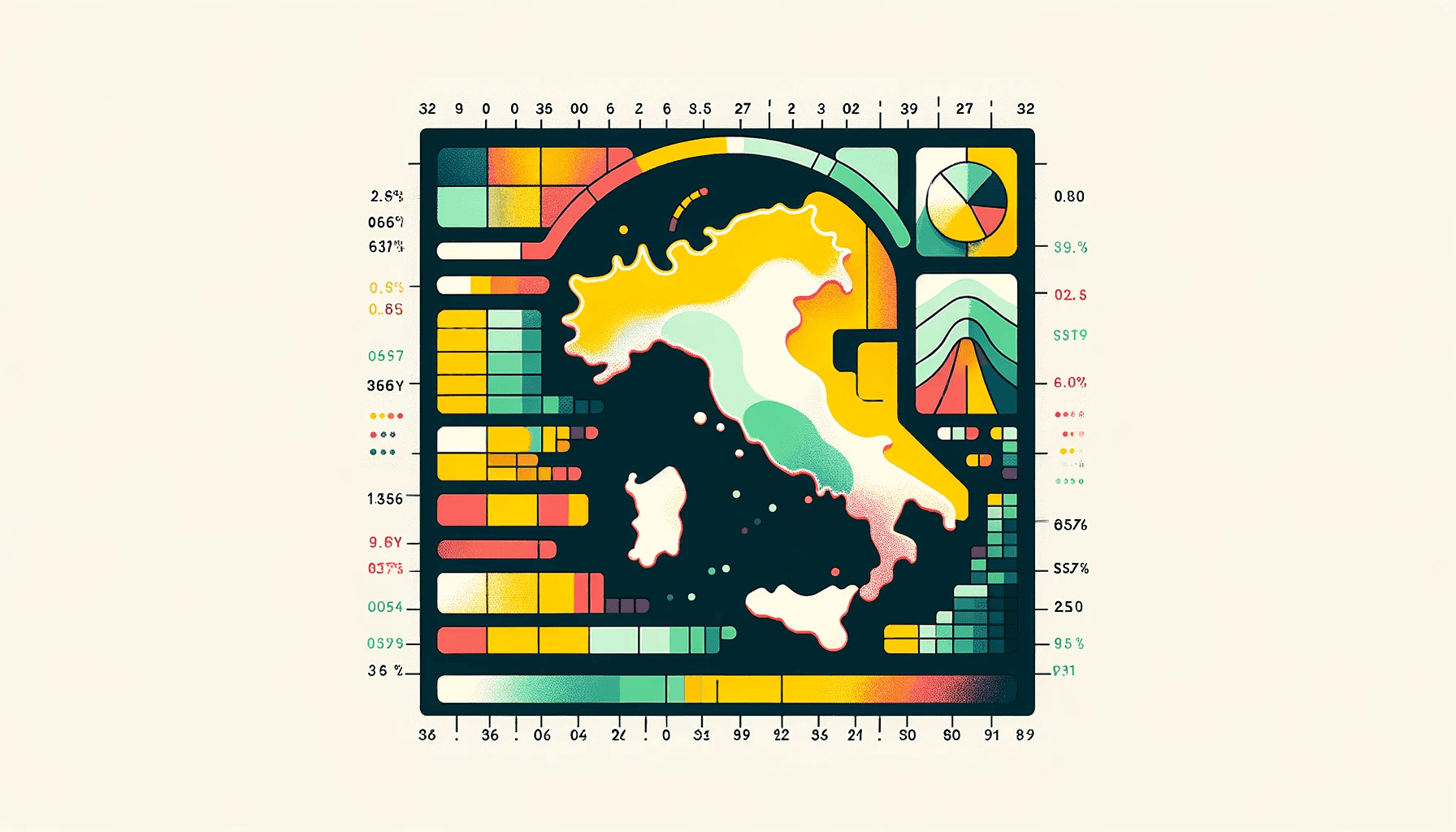
Choropleths are thematic maps where areas are shaded or patterned in proportion to the measurement of a statistical variable being displayed on the map, such as population density or per-capita income. In this tutorial, we will learn how to use the folium.colormap
module to enhance our choropleths with various color schemes.
First, make sure you have Folium installed, and verify its version:
import folium
print(folium.__version__)
If we attempt to load geographical and unemployment data for the U.S. states, we might encounter a file loading error if the data is not properly located. Assuming we have the us-states.json
and US_Unemployment_Oct2012.csv
in our data directory, we would load the data like this:
import json
import pandas as pd
import os
us_states = os.path.join('data', 'us-states.json')
US_Unemployment_Oct2012 = os.path.join('data', 'US_Unemployment_Oct2012.csv')
geo_json_data = json.load(open(us_states))
unemployment = pd.read_csv(US_Unemployment_Oct2012)
unemployment_dict = unemployment.set_index('State')['Unemployment']
self-defined color functions
You can create a choropleth using a custom function to determine colors. The function will map low values to one color (like green) and high values to another color (like red), for example:
def my_color_function(feature):
"""Maps low values to green and high values to red."""
if unemployment_dict[feature['id']] > 6.5:
return '#ff0000'
else:
return '#008000'
Using StepColormap
The StepColormap
can define colors and the corresponding thresholds:
import branca.colormap as cm
step = cm.StepColormap(['green', 'yellow', 'red'], vmin=3, vmax=10, index=[3, 4, 8, 10], caption='step')
step
Using LinearColormap
for continuous colors
If smooth transitions between colors are preferred, LinearColormap
could be used:
linear = cm.LinearColormap(['green', 'yellow', 'red'], vmin=3, vmax=10)
linear
To apply a colormap to a map, use the style_function
parameter when creating a GeoJson
object:
m = folium.Map(location=[43, -100], tiles='cartodbpositron', zoom_start=4)
folium.GeoJson(
geo_json_data,
style_function=lambda feature: {
'fillColor': linear(unemployment_dict[feature['id']]),
'color': 'black',
'weight': 2,
'dashArray': '5, 5'
}
).add_to(m)
m
Additional colormap features
You can also convert a LinearColormap
to a StepColormap
or vice versa, tweak built-in colormaps, and scale them to your dataset:
# Example of converting a LinearColormap to a StepColormap
linear.to_step(6)
# Using a built-in colormap
cm.linear.OrRd
# Scaling and converting a built-in colormap
cm.linear.YlGn.scale(3, 12).to_step(10)
Drawing a Colormap
on a map
A Colormap
can also be added directly to a Folium map:
m = folium.Map(tiles='cartodbpositron')
colormap = cm.linear.Set1.scale(0, 35).to_step(10)
colormap.caption = 'A colormap caption'
m.add_child(colormap)
m
This tutorial should provide a good starting point for creating visually appealing choropleths in Folium. Remember to save your map as an HTML file to view it in a web browser:
# Uncomment and run to save the map to a file
# m.save(os.path.join('results', 'Colormaps_3.html'))
Happy Mapping!
Summary
In short, we have covered how to use Folium's colormap
module to assign colors to choropleth maps effectively. We discussed self-defined color functions for discrete color mapping, StepColormap
and LinearColormap
for providing threshold-based and continuous color gradients, respectively. Furthermore, we learned about converting between linear and step colormaps, using built-in color scales, and how to draw a colormap legend directly on a Folium map. This skill set is a powerful addition to any data scientist or analyst's toolkit when visualizing geospatial data.