How to implement interactive and animated figures in Jupyter Notebooks
By Deepnote team
•Updated on November 23, 2023
This guide teaches how to enhance data presentations by implementing interactive and animated figures in Jupyter Notebooks.
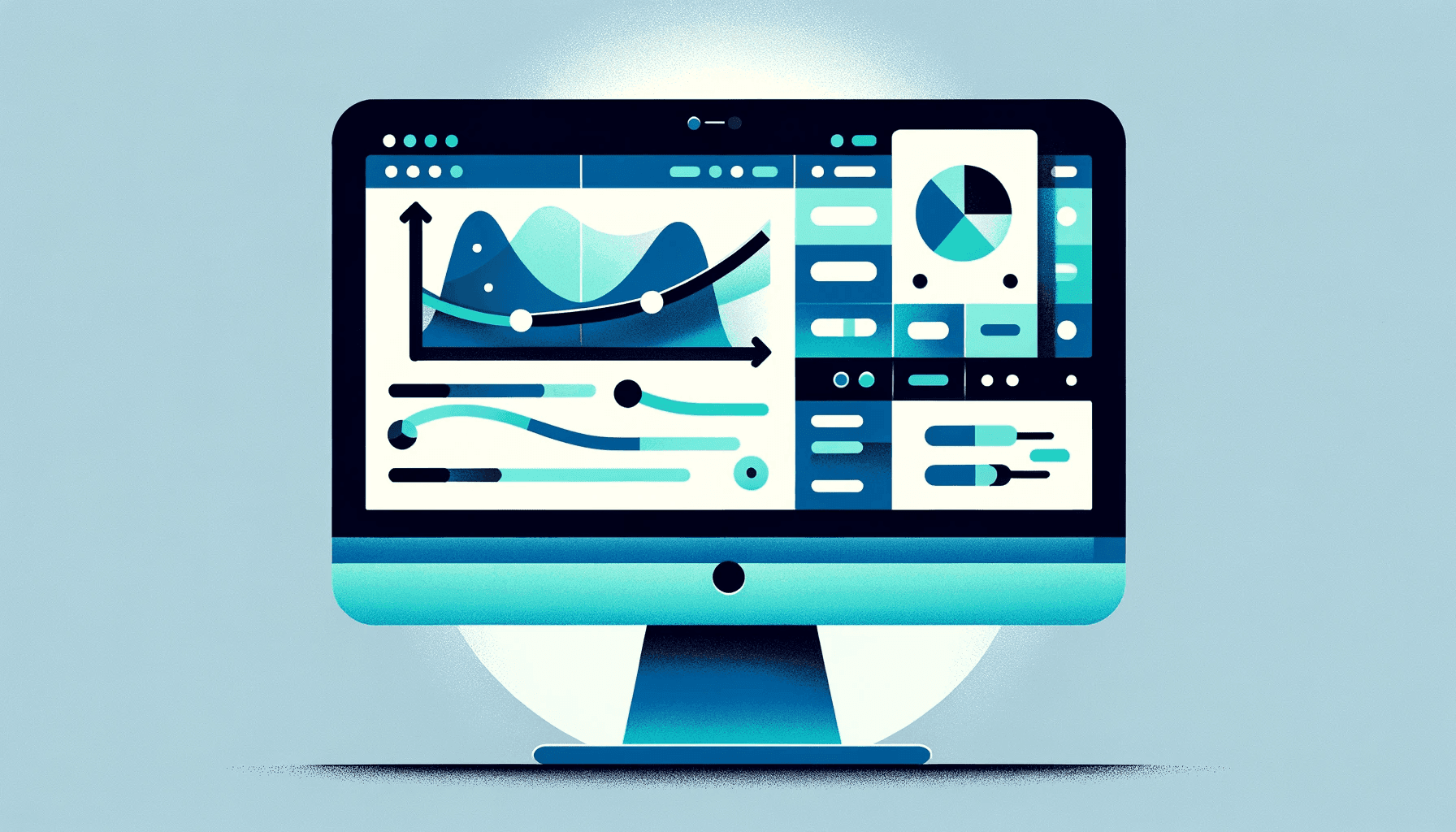
Jupyter Notebooks provide a versatile platform for data scientists and analysts to create interactive and animated figures. These capabilities enhance the educational and explanatory power of notebooks, allowing for dynamic visualization of concepts and data. In this tutorial, we'll delve into how to use Jupyter code cells to implement such figures.
How to create interactive plots with the FloatSlider widget
Interactive plots are an excellent way to explore data and mathematical functions dynamically. In Jupyter, you can create an interactive plot with the help of the ipywidgets
module. Consider a simple example where you want to visualize the graph of ( y = \cos(x + \phi) ) with a slider to adjust the value of ( \phi ). This is how you can do it:
-
Setup: Begin by setting up inline plotting with
%matplotlib inline
and import necessary modules:scipy
,matplotlib.pyplot
, andipywidgets
.%matplotlib inline from scipy import * import matplotlib.pyplot as plt from ipywidgets import interact, FloatSlider
-
Defining the Callback Function: Create a function, say
plot_cos
, that generates the plot. This function should take the slider value (in this case,phi
) as an argument.def plot_cos(phi): # Your plotting code here
-
Creating the Interactive UI: Use the
interact
function fromipywidgets
, passing your callback function and aFloatSlider
to create an interactive slider.interact(plot_cos, phi=FloatSlider(min=-3.2, max=3.2, step=0.2, value=0.0));
How to create 3D plots
3D plotting in Jupyter is enabled by mpl_toolkits.mplot3d
. Here's a brief guide:
-
Import Axes3D: Start by importing
Axes3D
frommpl_toolkits.mplot3d
.from mpl_toolkits.mplot3d import Axes3D
-
Initialize 3D Plot: Use
add_subplot
withprojection='3d'
to initialize a 3D plot.ax = fig.add_subplot(1, 1, 1, projection='3d')
-
Plotting in 3D: Use the usual
plt.plot
for curves, and for surfaces, use methods likeplot_trisurf
.
How to plot HTML5 animations
Animating plots in Jupyter can be highly engaging. Here's a quick guide to create an HTML5 video animation:
-
Importing Animation Module: Import the necessary animation submodule from
matplotlib
.from matplotlib import animation, rc rc('animation', html='html5')
-
Creating an Animation Function: Define a function for your animation. Within this function, set up the plot, initialize the plot objects to be animated, and define the animation and initialization functions.
-
Creating the Animator: Use
animation.FuncAnimation
to create the animator object. -
Displaying the Animation: Return or display the animator object at the end of your function.
return animator
Summary
In this tutorial, we explored the creation of interactive and animated figures in Jupyter Notebooks. These techniques greatly enhance the visualization capabilities of your notebooks, making them more engaging and useful for data exploration, educational purposes, and presentations. Whether it's through interactive sliders, 3D visualizations, or dynamic animations, Jupyter Notebooks offer a flexible platform for creative data visualization.