How to use Python's statistics functions
By Deepnote team
•Updated on November 23, 2023
Understanding and applying Python's statistics functions.
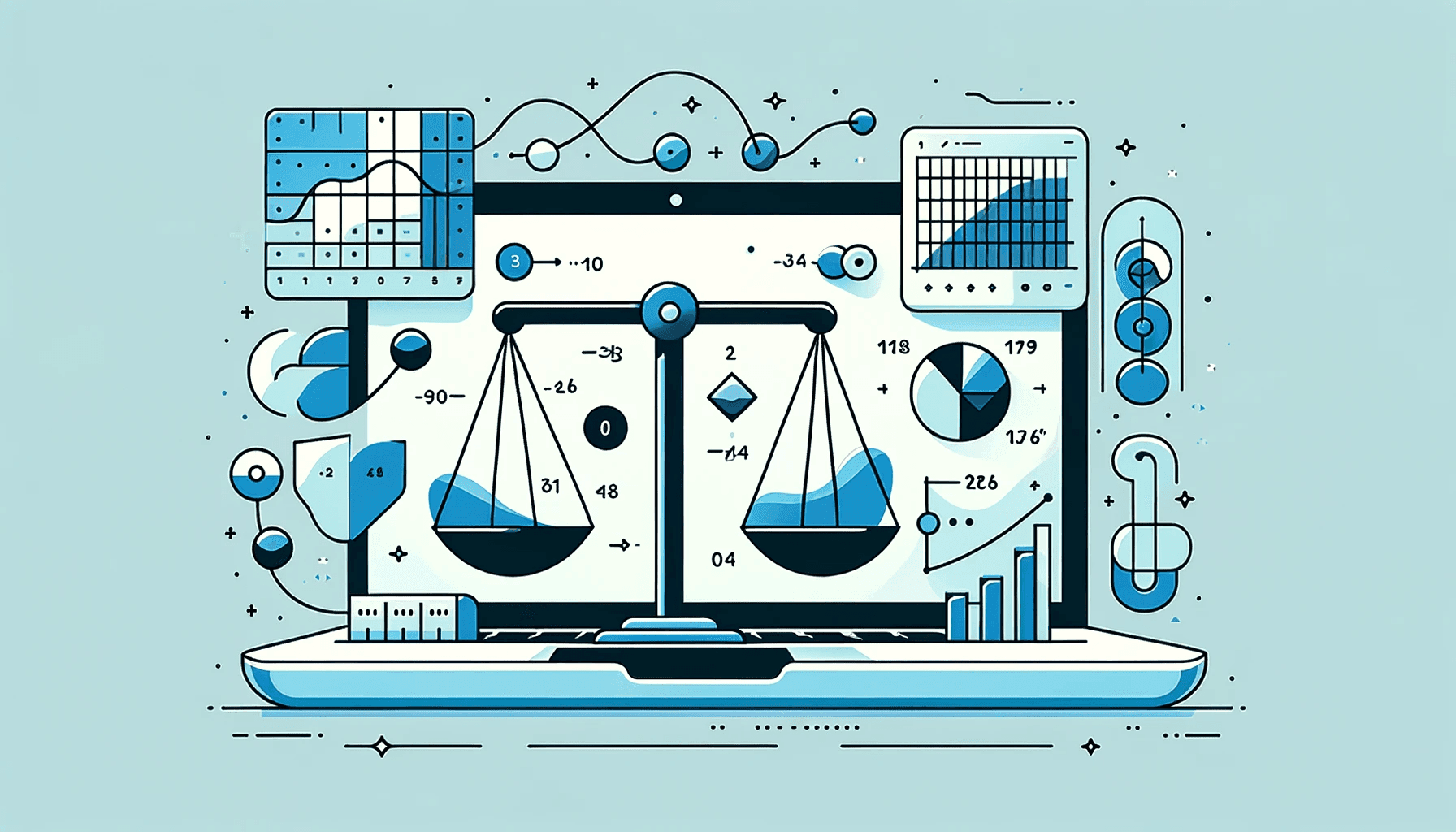
In this tutorial, we'll explore several useful statistics functions available in the Python Standard Library, specifically within the statistics
module. These functions are commonly employed in statistical analysis:
- mean: Calculates the average value.
- median: Determines the middle value in a dataset.
- mode: Identifies the most frequently occurring value.
- standard deviation: Measures the spread of values in a dataset.
How to import statistics functions in Python
To utilize these functions, you must first import them from the statistics
module as follows:
from statistics import mean, median, mode, stdev
Once imported, you can call mean()
, median()
, mode()
, and stdev()
directly. Since the statistics
module is included in the Python Standard Library, no additional package installations are necessary.
How to define a dataset in Python
Consider a dataset comprising five test scores: 60, 83, 83, 91, and 100. In Python, this data can be stored in a list, which is defined using square brackets []
and separates elements with commas:
test_scores = [60, 83, 83, 91, 100]
How to calculate the mean
To compute the mean or average of our test scores, apply the mean()
function from the statistics module:
average_score = mean(test_scores)
The result, average_score
, will be 83.4
.
How to calculate the median
The median, or middle value of the dataset, is obtained using the median()
function. For an odd number of values, it returns the middle value; for an even number, it returns the average of the two middle values:
median_score = median(test_scores)
Here, median_score
will be 83
.
How to calculate the mode
The mode, or most frequently occurring value, is calculated using the mode()
function. Note that if more than one value is equally common, the function raises a StatisticsError
:
most_common_score = mode(test_scores)
In this case, most_common_score
is 83
.
How to calculate the standard deviation
Standard deviation, indicating the spread of the data, is calculated with the stdev()
function. A larger standard deviation suggests more spread out data, while a smaller one indicates data clustered closely together:
score_spread = stdev(test_scores)
The score_spread
will be approximately 14.84
.
Alternative: Importing the entire statistics module
Alternatively, you can import the entire statistics
module:
import statistics
Then, use the functions with their full names, like statistics.mean(test_scores)
.
Summary
The statistics
module in Python provides handy functions for basic statistical calculations. You can import specific functions or the entire module depending on your needs. This module offers functions like mean()
, median()
, mode()
, and stdev()
, facilitating the computation of average, middle value, most common value, and data spread, respectively, in a given dataset.